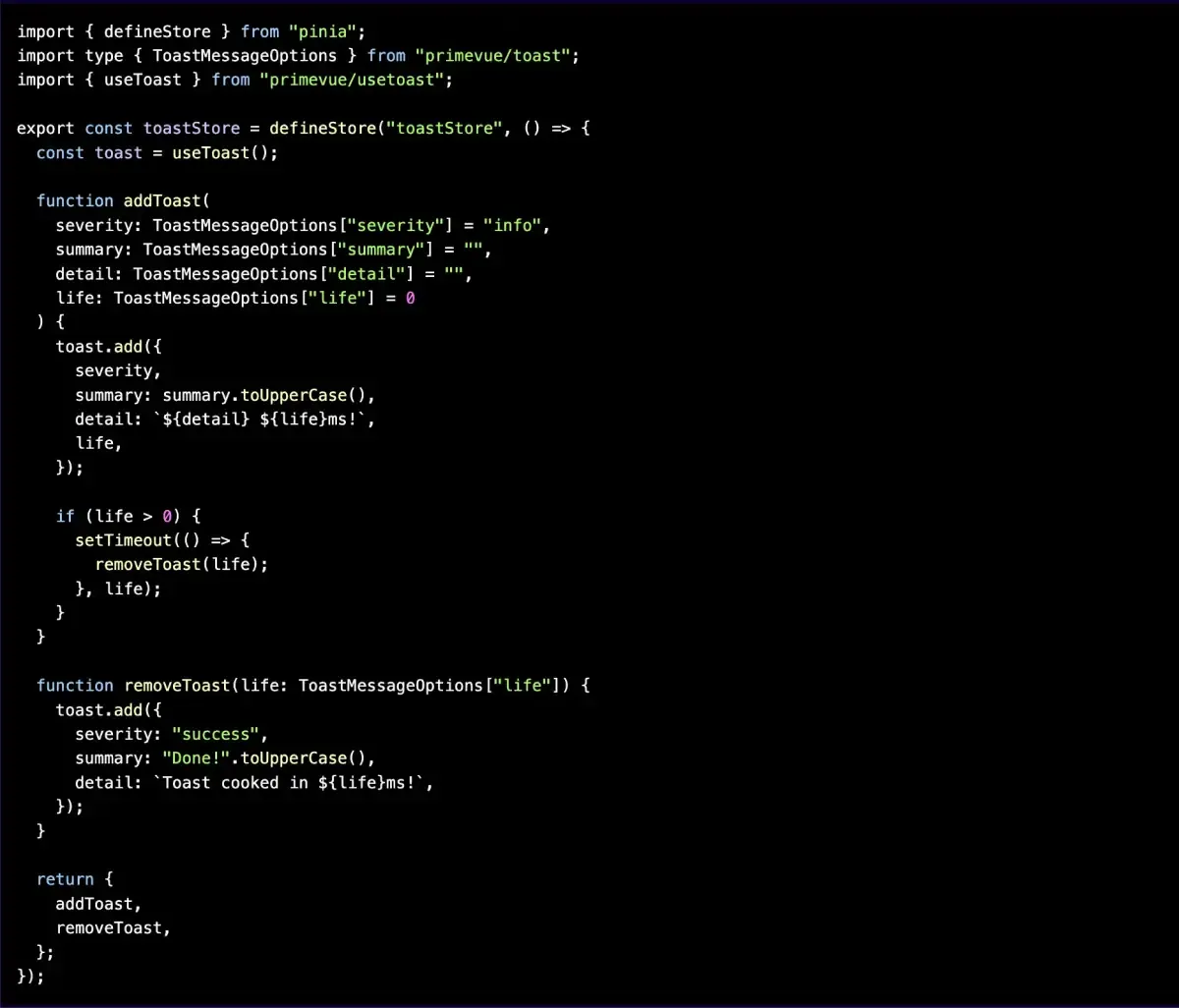
We're Having Toast: Toast Notifications in PrimeVue
Created: Friday, February 16, 2024 2:50 PMLast Updated: Friday, February 16, 2024 3:11 PM

Toast notifications are an essential component of modern web applications, providing users with timely feedback and alerts. In Vue.js applications, PrimeVue offers a convenient and customizable way to implement toast notifications through its Toast component. In this article, we'll explore how to use PrimeVue's toast notifications in Vue.js applications and examine a sample code snippet that demonstrates their usage.
import { defineStore } from "pinia"; import type { ToastMessageOptions } from "primevue/toast"; import { useToast } from "primevue/usetoast"; export const toastStore = defineStore("toastStore", () => { const toast = useToast(); function addToast( severity: ToastMessageOptions["severity"] = "info", summary: ToastMessageOptions["summary"] = "", detail: ToastMessageOptions["detail"] = "", life: ToastMessageOptions["life"] = 0 ) { toast.add({ severity, summary: summary.toUpperCase(), detail: `${detail} ${life}ms!`, life, }); if (life > 0) { setTimeout(() => { removeToast(life); }, life); } } function removeToast(life: ToastMessageOptions["life"]) { toast.add({ severity: "success", summary: "Done!".toUpperCase(), detail: `Toast cooked in ${life}ms!`, }); } return { addToast, removeToast }; });
Imports:
The code imports necessary functions and types from pinia and primevue libraries.
Store Definition:
toastStore is defined using defineStore from Pinia. This store will manage toast notifications in the application.
Toast Initialization:
useToast hook from PrimeVue is used to initialize the toast instance, which will be used to add and remove toast notifications.
Add Toast Function:
The addToast function creates a new toast with severity, summary, detail, and life duration, adding it with toast.add. For life > 0, a timeout auto-removes it.
Remove Toast Function:
The removeToast function is responsible for removing the toast notification. It adds a success toast indicating that the original toast has been successfully removed.
Return Object:
The store returns an object containing the addToast and removeToast functions, which can be accessed and used in Vue components.